Recently, I’ve been spending a lot of my limited free time trying to learn more about how to build blockchain applications on the ICON network. I don’t ever see myself being a full-blown developer, but I hope to gain enough knowledge and skills to be able to work with developers to build dApps on ICON.
I really like serverless functions because it allows me to run bits of code on demand without having to spin up and set up an entire server. For example, I use a serverless function to clear my Cloudflare cache on this blog after each deployment. As part of a small blockchain stats project I’m working on, I decided to use serverless functions to query data from the ICON blockchain.
In this post, I’ll show you how to use Google Cloud Functions to get details about the most recent block on the ICON blockchain with the Python SDK.
First, create a new function in Google Cloud Functions.
- Give the function a name. I used
get_latest_block
. - For the memory allocation, 128 MB is sufficient for a basic function.
- Set the “trigger” option to HTTP. This allows the function to be accessible via a URL like
https://region-username.cloudfunctions.net/function
. - Check “allow unauthenticated invocations” to make the function publicly accessible. This option is useful for creating public APIs.
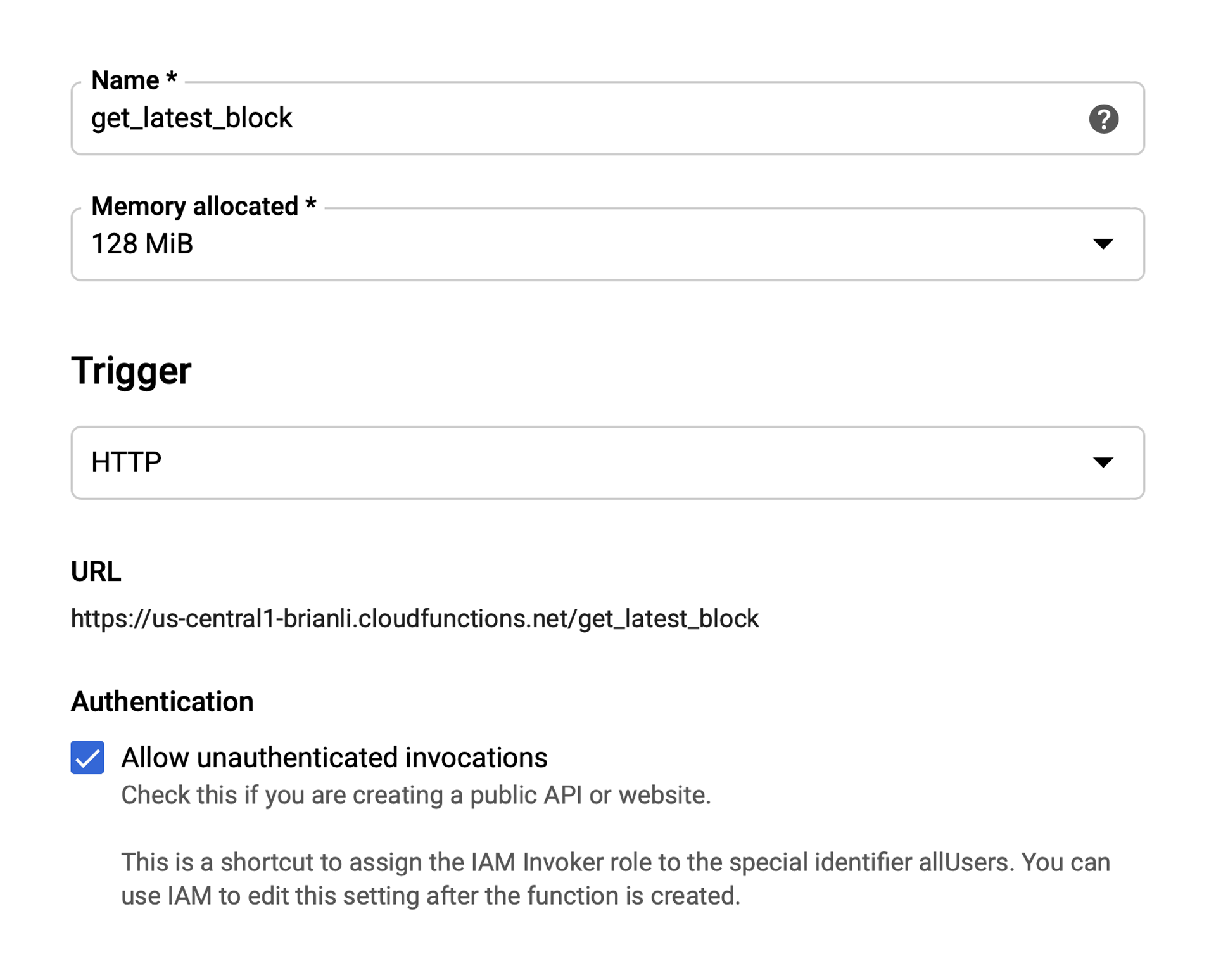
In the “source code” section, there a few ways to input the function code. Since we are writing such a basic function, the inline editor is fine.
- For the “runtime”, select
Python 3.7
. Google Cloud Functions supports a variety of runtimes, so feel free to select another one if you want.
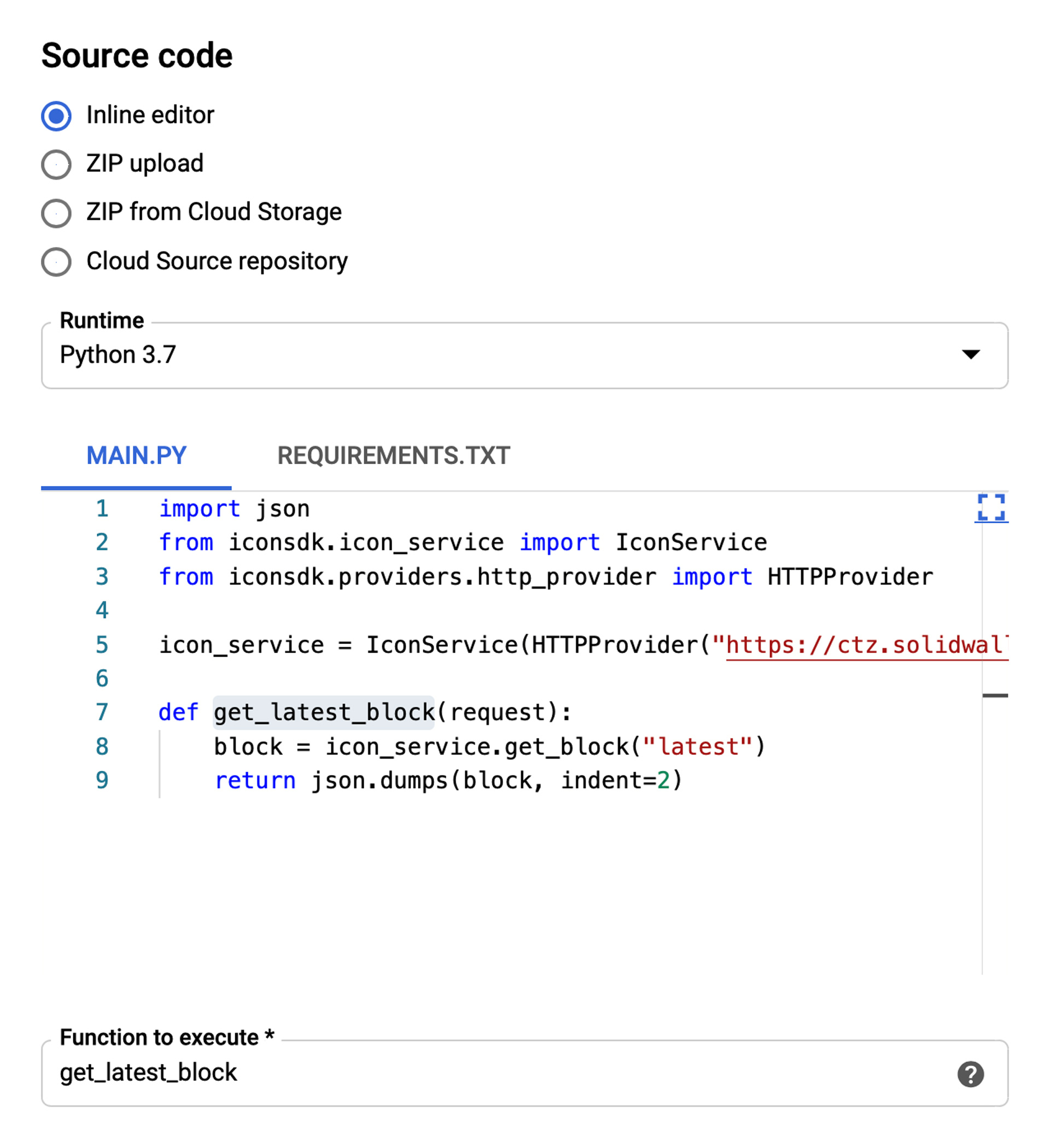
Next, let’s write the function for “MAIN.PY”. The code below contains a get_latest_block()
function. It connects to an ICON citizen node – https://ctz.solidwallet.io
– and makes a request to the ICON blockchain, and returns a JSON-formatted representation of the details in the latest block.
Notice that the function includes a request
argument. This argument is required for Google Cloud Functions using the HTTP trigger method.
import json
from iconsdk.icon_service import IconService
from iconsdk.providers.http_provider import HTTPProvider
icon_service = IconService(HTTPProvider("https://ctz.solidwallet.io", 3))
def get_latest_block(request):
block = icon_service.get_block("latest")
return json.dumps(block, indent=2)
After adding the code into the inline editor, click on “REQUIREMENTS.TXT”. This is where dependencies need to be added. For the function above, the iconsdk
library should be added as a dependency. The json
library is included in Python as a standard library, so it does not need to be added as a dependency.
The “REQUIREMENTS.TXT” text field should look like this.
## Function dependencies, for example:
## package>=version
iconsdk
Finally, specify get_latest_block
as the “function to execute”.
There are additional options for networking and environment variables, but we don’t need to configure these settings for this basic function.
Click the “Create” button to finalize the function.
The function will take a few minutes to deploy. After it has been deployed, click on the “Trigger” and visit the trigger URL.
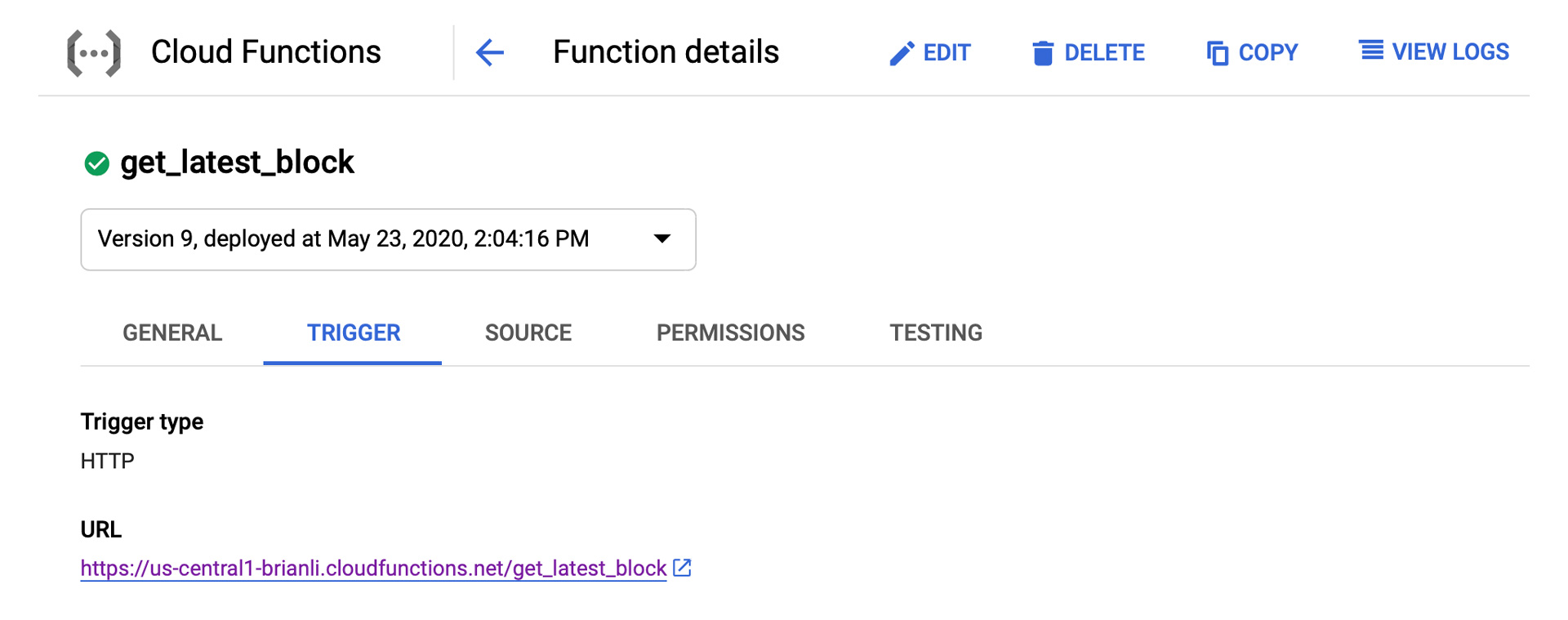
There may be a slight delay on the first invocation of the function, but subsequent requests will be very fast. If you set up everything correctly, you should see the latest block details in your browser window.
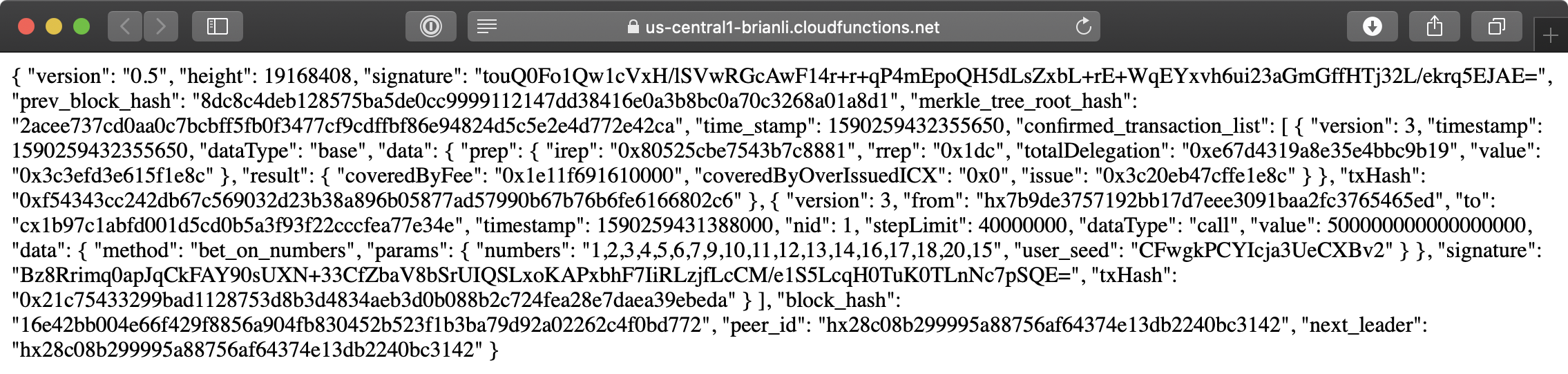
If you’re not familiar with how to deploy a server environment, using serverless functions to build out blockchain microservices for an API is a practical alternative. I hope you found this tutorial helpful, and feel free to reach out via Twitter or email if you have any questions or comments.